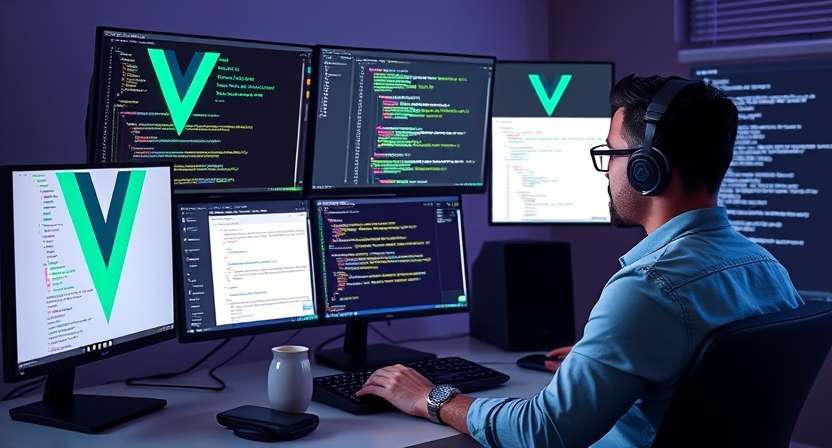
Introduction
Vue.js isn’t simply a structure for fledglings â it’s likewise an integral asset for proficient engineers constructing superior execution web applications. With its secluded engineering, high level elements, and vigorous biological system, Vue.js is intended to scale and adjust to complex application necessities.
This guide digs into cutting edge Vue.js ideas, best practices, and apparatuses to take your advancement abilities to a higher level. By dominating these, you’ll be prepared to construct modern, viable, and adaptable applications.
Key Features of Advanced Vue.js Development
- State Management: Centralized control with Vuex or Pinia.
- Server-Side Rendering (SSR): Enhanced performance and SEO with Nuxt.js.
- Composition API: Modern, flexible approach to handling complex logic.
- Testing and Debugging: Robust tools like Vue Test Utils and Vue DevTools.
- Integration with Modern Tools: Seamless use with TypeScript, GraphQL, and more.
Core Concepts for Professional Developers
- State Management with Vuex or Pinia
For large-scale applications, managing shared state is crucial. Vuex and Pinia provide centralized state management, making data flow predictable and manageable.- Vuex example:javascriptCopy code
const store = Vuex.createStore({ state: { count: 0 }, mutations: { increment(state) { state.count++; } } }); app.use(store);
- Vuex example:javascriptCopy code
- Optimizing Performance with SSR and Nuxt.js
Server-Side Rendering (SSR) improves app performance and SEO. Nuxt.js, built on Vue, simplifies SSR implementation.- Install Nuxt.js:bashCopy code
npx create-nuxt-app my-app
- Install Nuxt.js:bashCopy code
- Implementing the Composition API
The Composition API organizes logic more flexibly compared to the Options API.- Example:javascriptCopy code
import { ref } from 'vue'; export default { setup() { const count = ref(0); const increment = () => count.value++; return { count, increment }; } };
- Example:javascriptCopy code
- Testing with Vue Test Utils
Unit and integration tests are essential for professional development. Vue Test Utils simplifies testing Vue components.- Example:javascriptCopy code
import { mount } from '@vue/test-utils'; import MyComponent from './MyComponent.vue'; test('renders props.message when passed', () => { const wrapper = mount(MyComponent, { props: { message: 'Hello Vue!' } }); expect(wrapper.text()).toContain('Hello Vue!'); });
- Example:javascriptCopy code
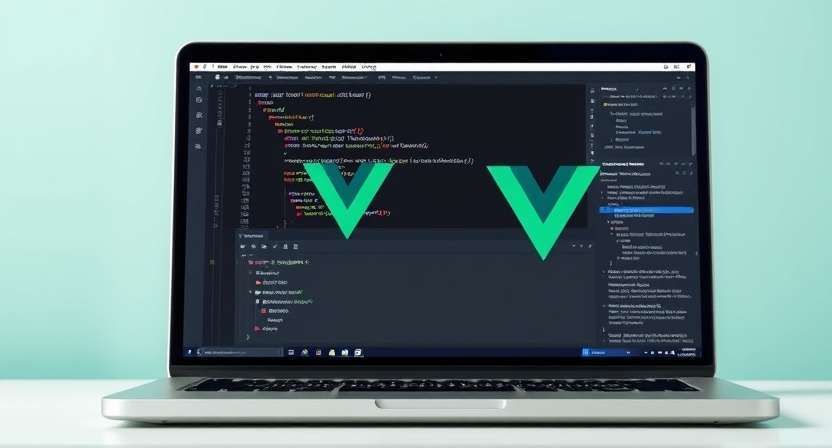
Advanced Best Practices for Vue.js
- Component Design: Create reusable and modular components to simplify maintenance.
- Dynamic Routing: Use Vue Router to manage complex navigation.
- Error Handling: Implement global error handlers for consistent error responses.
- Code Splitting: Load components on demand to optimize performance.
FAQs About Professional Vue.js Development
Q1: When should I switch from the Options API to the Composition API?
The Composition API is ideal for large projects with complex logic. If your code feels unwieldy with the Options API, consider switching.
Q2: What are the benefits of using Nuxt.js?
Nuxt.js simplifies SSR, enhances SEO, and provides out-of-the-box features like routing and state management.
Q3: How does TypeScript integrate with Vue.js?
Vue 3 has excellent TypeScript support, allowing you to write type-safe code for better maintainability.
Q4: Is Vue.js suitable for enterprise-level applications?
Yes! Vue.jsâs scalability, performance, and robust ecosystem make it suitable for large, enterprise-grade applications.
Q5: How do I keep my Vue.js skills up to date?
Follow the Vue.js documentation, participate in forums, and explore new plugins and tools to stay current.
Conclusion
Mastering Vue.js at an expert level opens vast opportunities for making present day, high-performing web applications. By utilizing state the board, SSR, and best practices, you can plan adaptable and viable applications that stick out.
Whether you’re building SPAs, incorporating complex APIs, or upgrading execution, Vue.js gives the apparatuses you really want. Continue refining your abilities, trying different things with cutting edge ideas, and investigating the tremendous Vue.js biological system to succeed in your advancement process.